Params & Body
new data.json
{
"users": [
{
"id": 1,
"firstname": "Alice",
"balance": 1000,
"credit_ID": 2
},
{
"id": 2,
"firstname": "Bob",
"balance": 500,
"credit_ID": 1
},
{
"id": 3,
"firstname": "Charlie",
"balance": 1500,
"credit_ID": 4
},
{
"id": 4,
"firstname": "David",
"balance": 2000,
"credit_ID": 3
}
],
"banks": [
{
"id": 1,
"owner": 1,
"sender": "Alice",
"receiver": 2,
"note": "For lunch",
"amount": 200,
"bank": "Bank A",
"type": "transfer"
},
{
"id": 2,
"owner": 2,
"sender": "Bob",
"receiver": 1,
"note": "Repayment",
"amount": 100,
"bank": "Bank B",
"type": "transfer"
},
{
"id": 3,
"owner": 3,
"sender": "Charlie",
"receiver": 4,
"note": "Gift",
"amount": 500,
"bank": "Bank C",
"type": "transfer"
},
{
"id": 4,
"owner": 1,
"sender": "Alice",
"receiver": 3,
"note": "For lunch",
"amount": 500,
"bank": "Bank A",
"type": "transfer"
},
{
"id": 5,
"owner": 1,
"sender": "Alice",
"receiver": null,
"note": "Dispose for expenses",
"amount": 300,
"bank": "Bank A",
"type": "dispose"
},
{
"id": 6,
"owner": 3,
"sender": null,
"receiver": "Charlie",
"note": "Withdraw for emergency",
"amount": 200,
"bank": "Bank C",
"type": "withdraw"
},
{
"id": 7,
"owner": 2,
"sender": null,
"receiver": "Bob",
"note": "Withdraw for rent",
"amount": 150,
"bank": "Bank B",
"type": "withdraw"
},
{
"id": 8,
"owner": 4,
"sender": null,
"receiver": "David",
"note": "Dispose for savings",
"amount": 1000,
"bank": "Bank C",
"type": "dispose"
},
{
"id": 9,
"owner": 4,
"sender": null,
"receiver": "David",
"note": "Withdraw for investment",
"amount": 700,
"bank": "Bank C",
"type": "withdraw"
},
{
"id": 10,
"owner": 2,
"sender": null,
"receiver": "Bob",
"note": "Dispose for utilities",
"amount": 80,
"bank": "Bank B",
"type": "dispose"
}
]
}
In JavaScript, "Params," "Query," and "Body" typically refer to different parts of an HTTP request when building web applications, especially when working with frameworks like Express.js in Node.js.
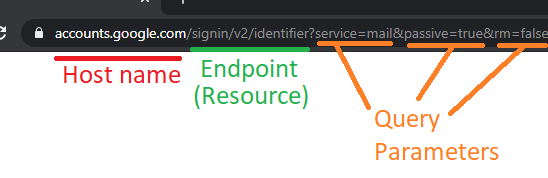
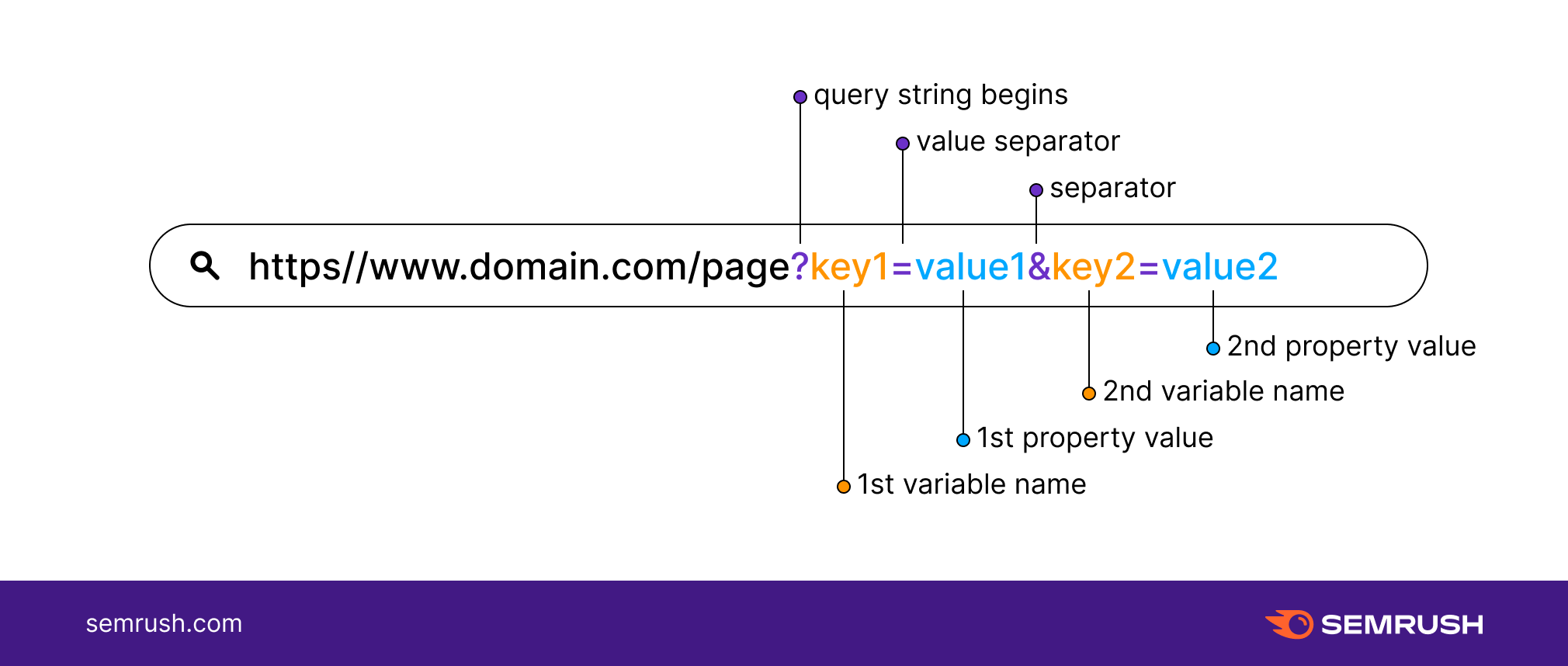
Params: These are URL parameters passed in the URL itself. They are part of the URL path and typically used to identify a specific resource. For example, in the URL /users/:userId
, :userId
is a parameter that can be accessed in the server-side code.
Example:
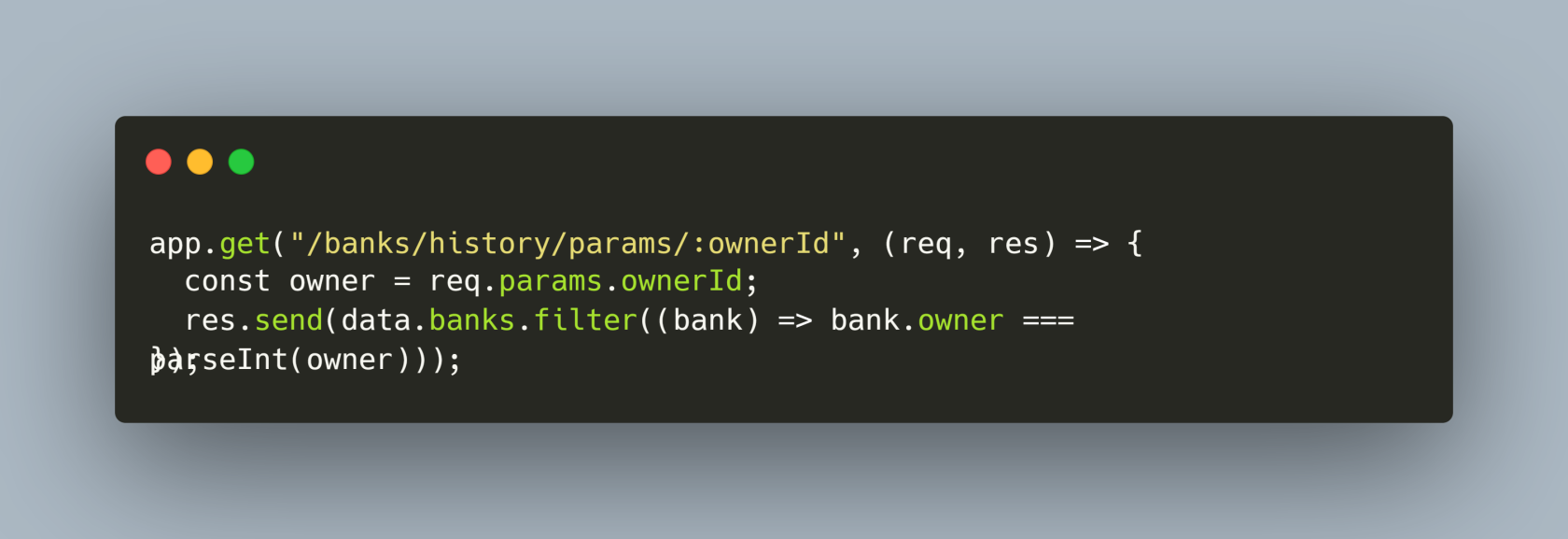
Query: These are parameters passed in the URL query string. They come after the ?
in a URL and are used for filtering or modifying the resource being requested.
Example:
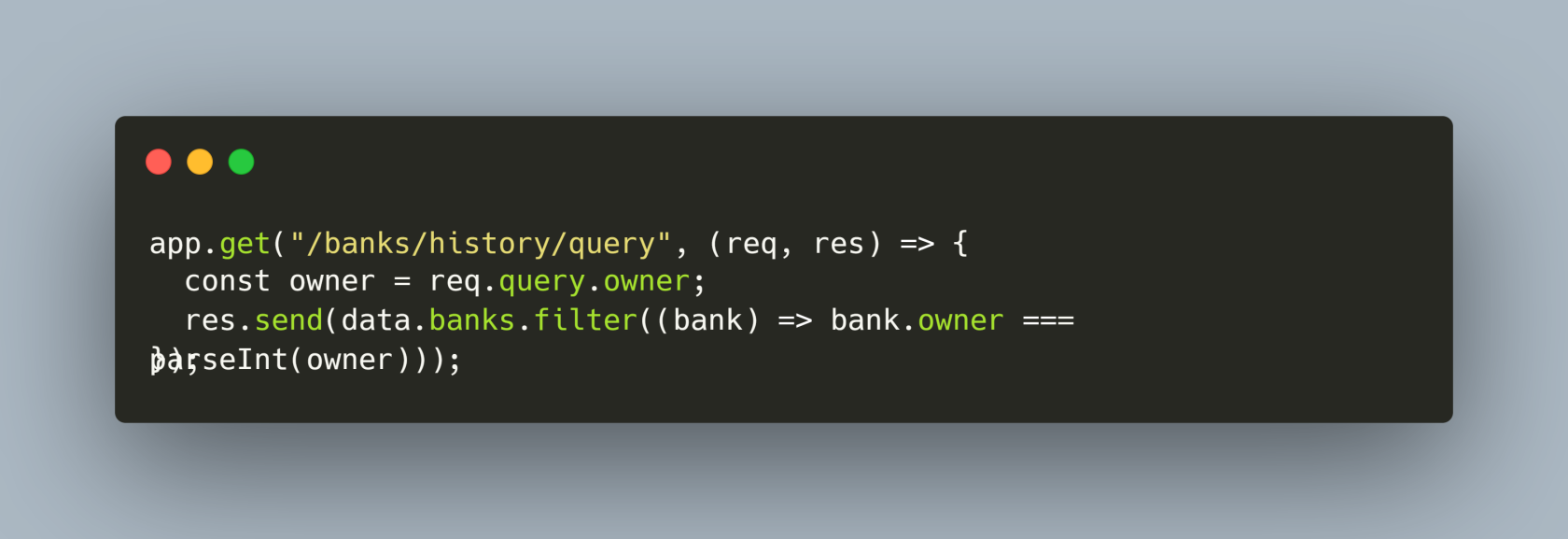
Body: This is the data sent as part of the HTTP request body. It is used to send more complex data, like JSON objects, from the client to the server, typically in POST or PUT requests.
To access the body in Express.js, you typically need middleware like body-parser
to parse the body of the request. In more modern versions of Express.js, this functionality is integrated into Express itself.
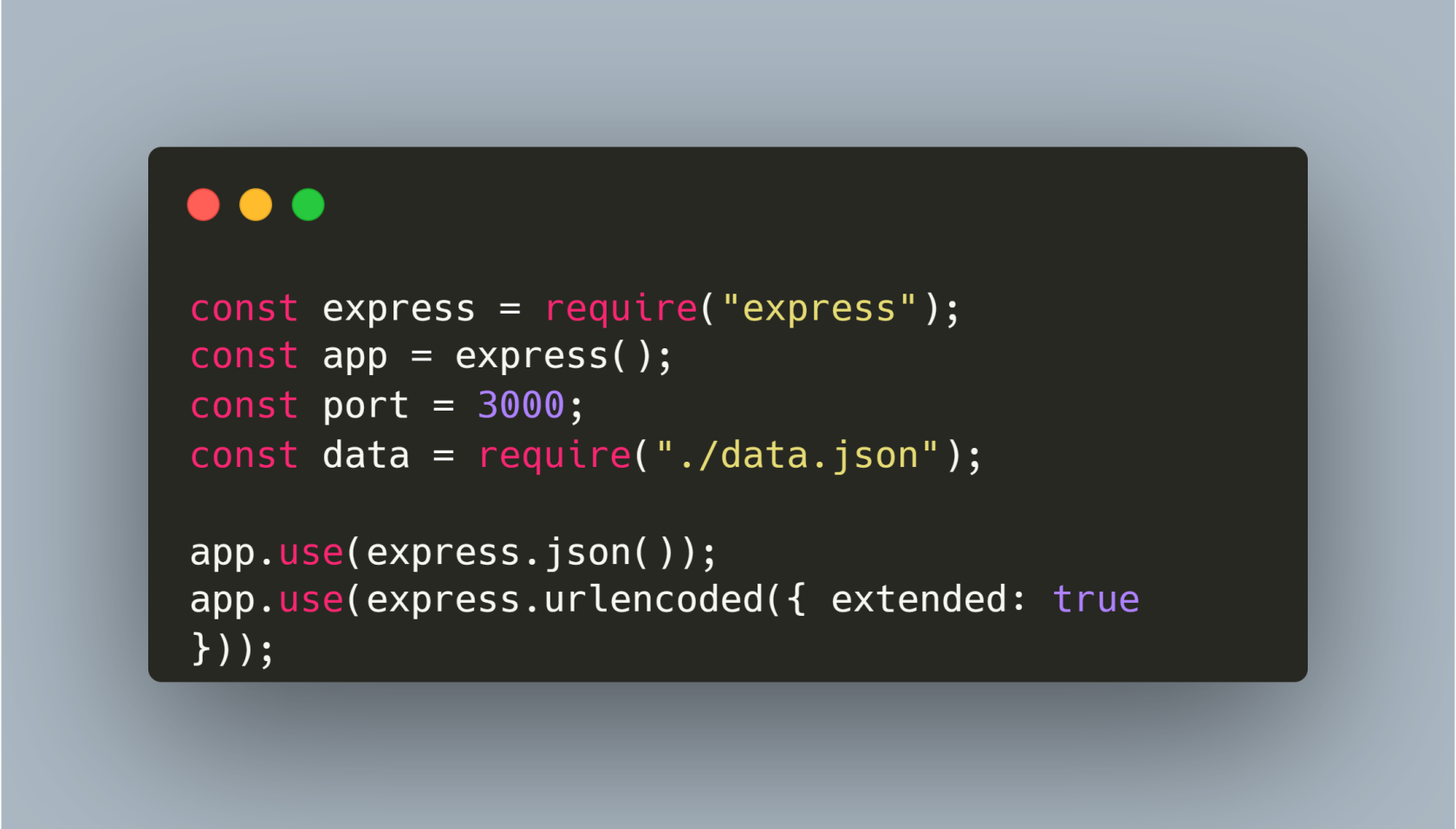
Example:
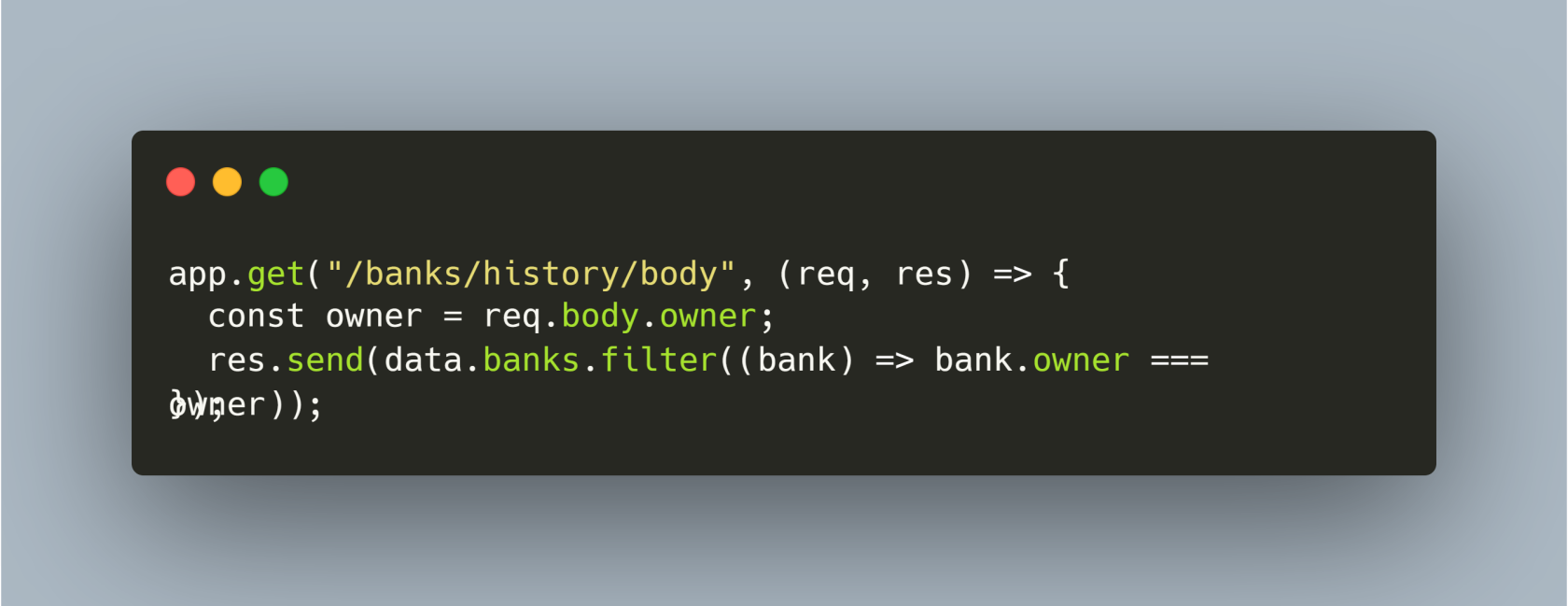
To Testing send body using the postman
- open postman and click body

- click raw
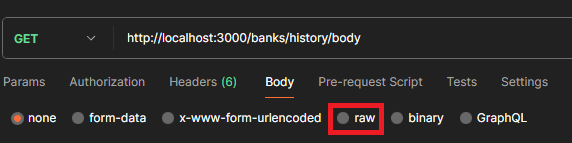
- select sending type JSON
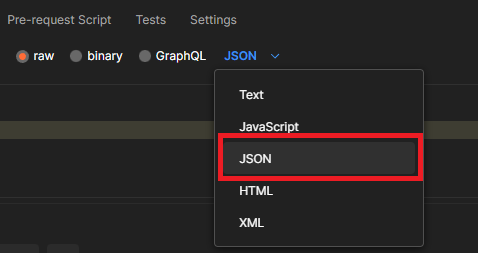
- fill the Body
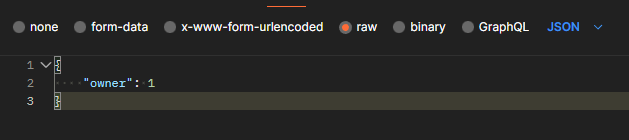
- send
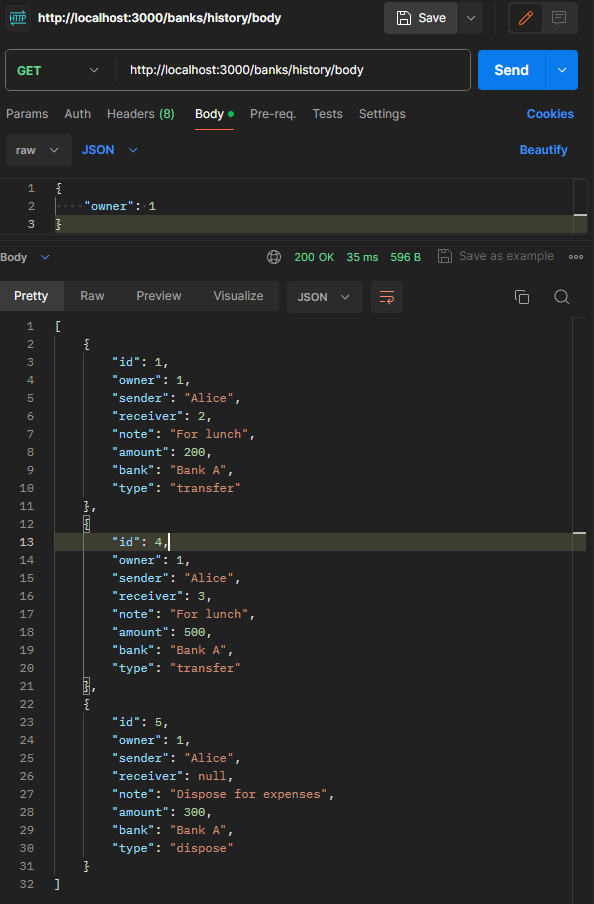
-- End of this example --